Learn how blockchain truly works, master key definitions, and uncover what makes smart contracts so "smart." Dive into the fundamentals, gain valuable insights, and start your blockchain journey today!
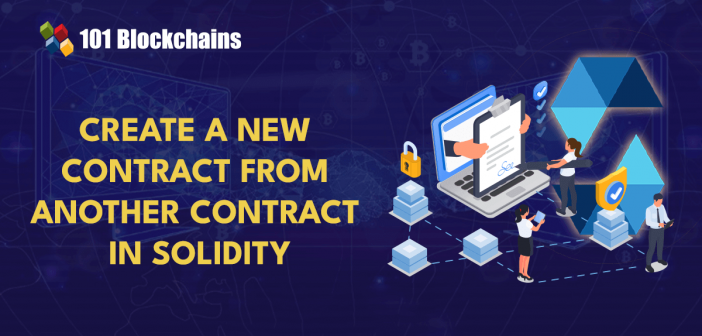
- Solidity & Smart Contracts
James Howell
- on August 03, 2023
How to Create a New Contract from another Contract in Solidity?
Smart contracts serve as the foundation of a continuously expanding blockchain ecosystem. You can find multiple use cases of blockchain in different industries which rely on smart contracts. For example, smart contracts help in storing the proof of ownership of NFTs on a blockchain network. The most popular programming language for creating smart contracts, Solidity, offers multiple features for smart contract development.
However, it is important to learn about the different ways to create a smart contract using a solidity programming language. Did you know that you can create a contract from another contract in Solidity? The following post offers you a detailed understanding of the process of creating a contract from another contract in Solidity.
Curious to understand the complete smart contract development lifecycle? Enroll now in Smart Contracts Development Course
How Can You Create Smart Contracts in Solidity?
Solidity offers two distinct options for creating a smart contract. A developer or ‘creator’ could develop the smart contract externally and deploy it, or a user interacting with a dApp that helps in deployment of smart contracts. Another option is to create contract from contract in Solidity with the help of a factory pattern. It involves the creation of different instances of one smart contract. Let us learn more about the factory pattern and its importance.
What is the Need for Factory Pattern?
The advantages of innovation with blockchain technology are one of the reasons for attracting users. On the other hand, the cost of mining data on blockchain is expensive. Users have to pay fees for every new block mined on blockchain networks. If you want to deploy smart contracts on blockchain, it will mine the contract data to the next block. In the case of Ethereum blockchain, you would have to pay gas fees in ETH for deploying smart contracts.
Build your identity as a certified blockchain expert with 101 Blockchains’ Blockchain Certifications designed to provide enhanced career prospects.
Definition of Factory Pattern
The responses to questions such as “How do you inherit two contracts in Solidity?” would refer to the factory design pattern. A factory design pattern is a popular programming concept that involves direct creation of a single object, known as the factory. It helps in direct creation of object instances for the developer.
In the case of Solidity, you can view smart contracts as objects, and a factory in Solidity could be smart contracts that could deploy different instances for other contracts. Developers could have to generate different objects for various use cases. However, it is difficult to find out the type of object you have to instantiate before the runtime execution of code. The factory pattern technique offers promising advantages for resolving such issues.
The best example to understand how to deploy contract from contract Solidity programming is a basic factory contract. You can rely on a simple factory contract for deploying different instances of one smart contract. In addition, you can also store the new instances on blockchain and access them upon requirement. Developers can also introduce new functionalities for managing the newly deployed contracts through a particular instance of the smart contract. In addition, developers can also add other functionalities, such as disabling the contract instance.
Are you aspiring to learn the fundamentals of the Ethereum Virtual Machine and smart contracts’ upgradability? Enroll now in the Advanced Solidity Development Course.
Advantages of Factory Pattern
The definition of factory pattern in programming and smart contract development showcases its potential for improving smart contract development. Smart contracts in Solidity call another contract with the help of a factory pattern, with one factory capable of offering new functionalities.
Most important of all, the factory design pattern helps in deploying a wide range of smart contracts with higher gas efficiency. You can maintain a tab on all the deployed contracts on blockchain networks with the help of factory contracts. One of the biggest advantages of deploying a contract from another contract in Solidity is the option of saving gas for different contract deployments.
A factory design pattern is useful to create contract from contract in special situations when you need multiple smart contract instances. It is also a promising tool for situations when you deal with various contracts that have similar functionalities.
Variants of Factory Design Patterns in Solidity
You can deploy contract from contract Solidity programming examples with their different variants. First of all, you can come across the normal factory pattern for Solidity contracts. The general factory pattern involves deploying multiple instances of multiple smart contracts without optimization for cost-effective deployment.
Another type of factory pattern in smart contracts with Solidity programming language refers to the cloned factory design pattern. The cloned factory pattern helps in deploying different instances of other contracts alongside focusing on optimization for saving gas costs in each deployment.
Want to get an in-depth understanding of Solidity concepts? Enroll now in Solidity Fundamentals Course
How to Create a Contract from another Contract in Solidity?
You can understand how to create a smart contract from another contract in Solidity by creating a simple contract. The factory contract would use the following simple contract for deploying its multiple instances.
// SPDX-License-Identifier: MIT pragma solidity >0.4.23 <0.9.0; contract Foundation { string public name; address public _owner; constructor( string memory _name, address _owner ) public { name = _name; _owner = msg.sender; } }
The first line of the contract in this example showcases the open-source license of the contract. It is important to note that Ethereum is an open-source project. The second line helps you move closer to answers for “How do you inherit two contracts in Solidity?” by specifying the Solidity version required for executing the contract.
In the next line, the logic establishes the Foundation smart contract, which is similar to classes in object-oriented programming languages. The constructor function, in this case, helps in initializing the state variables of the contract with values passed in the form of arguments. The constructor function is called upon the creation of an instance of the contract.
Excited to learn the basic and advanced concepts of ethereum technology? Enroll now in Ethereum Technology Course
Example of Writing a Factory Contract
The Foundation smart contract outlined in the example is to create contract from contract with Solidity programming language. On the other hand, it does not have the foundations for creation and deployment. Therefore, it is important to create a factory contract that could create individual instances of Foundation smart contracts by utilizing the normal factory contract pattern. Here is an example of a normal factory pattern smart contract.
// SPDX-License-Identifier: MIT pragma solidity >0.4.23 <0.9.0; import "./Foundation.sol"; contract FoundationFactory { Foundation[] private _foundations; function createFoundation( string memory name ) public { Foundation foundation = new Foundation( name, msg.sender ); _foundations.push(foundation); } function allFoundations(uint256 limit, uint256 offset) public view returns (Foundation[] memory coll) { return coll; } }
The function “createFoundation” in the factory design pattern code helps in deploying one instance of the Foundation smart contract. It also helps in storing the smart contract instance on the blockchain. On the other hand, the “allFoundations” function helps in retrieving different instances of Foundation contracts hosted on the blockchain network.
Using New Keywords for Creating a Contract from Other Smart Contracts
Factory design pattern serves as one of the most valuable concepts for creating smart contracts from another smart contract in Solidity. However, you can also use the Solidity new keyword for creating smart contracts from another Solidity contract. You can find out the way the ‘new’ keyword works in Solidity through a simple factory example.
Assume that you have a parent contract that would deploy another instance of a child contract. In this case, you have to create only one instance of a smart contract in Solidity from the parent contract. You don’t have to worry about having twins or triplets, as it could be more than you can handle. Developers can use the ‘new’ keyword for creating one or multiple instances of smart contracts according to your requirements.
Excited to learn about different examples of Solidity smart contract code and their significance, Read here What are the Best Solidity Smart Contract Examples?
Example of a Family with Smart Contracts and New Keyword
You have to take a parent contract, which you can refer to as DadContract, that would deploy a child contract, SonContract. Here is an example of the parent contract code.
pragma solidity ^0.5.0;import "./SonContract.sol";contract DadContract { string public name; uint public age; SonContract public son; constructor( string memory _dadsName, uint _dadsAge, string memory _sonsName, uint _sonsAge ) public { son = new SonContract(_sonsName, _sonsAge); name = _dadsName; age = _dadsAge; } }
The example to deploy contract from contract Solidity with the help of a new keyword begins with the ‘DadContract’ and creates the ‘SonContract.’ You should also pass the parameters required for creating the SonContract to the DadContract, following which it can pass them. The contract code also includes the ‘son’ variable of the type of ‘SonContract,’ which allows calling the contract. Here is an example of the code for the ‘SonContract.’
pragma solidity ^0.5.0;contract SonContract { string public name; uint public age; constructor( string memory _sonsName, uint _sonsAge ) public { name = _sonsName; age = _sonsAge; } }
The values for name and age passed from the DadContract help in creating the SonContract. In this example, you can notice that the arguments have been set on the ‘name’ and ‘age’ variables.
Methods to Call a Smart Contract from another Contract
Smart contracts are computer applications on a blockchain network that can run without the need for central parties or servers. Smart contracts can interact with the deployed contracts, which also applies to EVM-compatible blockchains. The responses to “How do you inherit two contracts in Solidity?” point to examples of different smart contracts which can interact with other contracts. Token issuers or minters can have permission to call the smart contract of a token. Similarly, decentralized exchanges could also interact with other smart contracts through the traded tokens.
Start your journey to become a smart contract developer or architect with an in-depth overview of smart contract fundamentals with Smart Contracts Skill Path
What are the Reasons for Calling a Function from another Smart Contract?
The situations in which you need to split an application into several contracts would require you to call a function from another smart contract. For example, you can create a smart contract using Solidity and divide it into three smart contracts which can interact with each other. Another notable use case for calling a function from another smart contract points to upgradeable contracts. Blockchain technology offers the advantage of immutability, which ensures that you cannot change the code of a smart contract after deployment. However, you can create a proxy that makes function calls to another contract containing the actual business logic.
People who need to change the logic can offer a different target address for the proxy contract, such as a new version with few improvements. In such cases, you can differentiate logic and data into separate smart contracts. The logic contract can be updated or swapped through a proxy contract, alongside retaining information in data contracts.
The option of using the Solidity new keyword to create a new contract from another contract offers powerful functionalities. You can use the proxy contract to enable the reuse of code and the flexibility for using contracts as libraries. It could also help in reducing deployment costs as the factory contract does not have to include all the codes of the contracts it creates.
Future of Creating New Contracts from another Contract in Solidity
You can think of the possibilities for using a factory pattern or the new keyword in Solidity. Developers can access functions of another smart contract by passing the address in a constructor of another contract. However, the integration of real-world functionalities such as multi-sig contracts can be a challenging task.
In addition, you would eventually reach a point where you could not introduce new functions as the smart contracts become large. The use of flexible methods for deploying multiple instances of smart contracts offers a promising solution to the cost of smart contract deployment.
Want to become a smart contract developer? Checkout detailed guide on How To Become A Smart Contract Developer
Conclusion
The guide to create contract from contract in Solidity programming language offers answers for the importance of using factory pattern. It showcases a unique programming concept that helps in creating multiple instances of objects directly. Considering the fact that smart contracts are also objects in an object-oriented programming language, developers can create multiple smart contract instances.
The factory contract can help in deploying different contracts according to the desired parameters. In addition, the Solidity new keyword is also an important highlight in the process of creating a smart contract with another smart contract. It can help you in creating one contract out of the factory contract, as you can notice in the DadContract and SonContract example. Learn more about Solidity fundamentals and explore Solidity docs for detailed procedures of creating smart contracts from another contract right now.
*Disclaimer: The article should not be taken as, and is not intended to provide any investment advice. Claims made in this article do not constitute investment advice and should not be taken as such. 101 Blockchains shall not be responsible for any loss sustained by any person who relies on this article. Do your own research!